Hello,
I'm creating a web application that will take user input from a javascript
map to give me bounding coordinates (i.e. urcrnrlat, urcrnrlon, llcrnrlat,
llcrnrlon) and possibly a switch for polar projection. Other than that I
have no further information. Which projection is the most suitable to handle
anything from a 'global' plot to a zoom say over a state? I don't see the
zoom being too tight, but global projections are likely. I personally prefer
Equal Area, hence right now I'm working with 'aeqd', but I seem to have
problems if the plot is global with that projection.
Just looking for advice, opinions, and ideally examples if anyone has
created a similar function / module to use in a web environment.
Thanks!
-john
···
--
View this message in context: http://www.nabble.com/dynamic-basemap-tp20121594p20121594.html
Sent from the matplotlib - users mailing list archive at Nabble.com.
John [H2O] wrote:
Hello,
I'm creating a web application that will take user input from a javascript
map to give me bounding coordinates (i.e. urcrnrlat, urcrnrlon, llcrnrlat,
llcrnrlon) and possibly a switch for polar projection. Other than that I
have no further information. Which projection is the most suitable to handle
anything from a 'global' plot to a zoom say over a state? I don't see the
zoom being too tight, but global projections are likely. I personally prefer
Equal Area, hence right now I'm working with 'aeqd', but I seem to have
problems if the plot is global with that projection.
Just looking for advice, opinions, and ideally examples if anyone has
created a similar function / module to use in a web environment.
Thanks!
-john
John: Sounds like you need one of the global cylindrical projections, such as cylindrical equidistant ('cyl'), miller ('mill') or mercator ('merc'). In the svn version, you also have Gall Stereographic ('gall'). See http://matplotlib.sourceforge.net/basemap/doc/html/users/mapsetup.html for examples. Unfortunately, none of them are equal-area. Mollweide ('moll') is a global equal-area projection, but it's only global (you can't specify a domain that isn't global). For the polar option, I suggest polar lambert azimuthal equal-area (http://matplotlib.sourceforge.net/basemap/doc/html/users/plaea.html). The user can specify whether he/she wants the south or north polar aspect, and the latitude of the outer edge.
-Jeff
···
--
Jeffrey S. Whitaker Phone : (303)497-6313
NOAA/OAR/CDC R/PSD1 FAX : (303)497-6449
325 Broadway Boulder, CO, USA 80305-3328
Hi,
I think I am running into the same thing John is here. When you want to
display the whole earth in 'aeqd' mode, the projection needs to be onto a
circle. As it is, what is plotted is a square that just fits inside the
circle I want. Here is a link to a photo of the kind of projection I want.
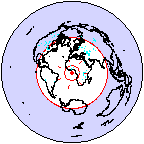
Is there a way to get the whole earth plotted with 'aeqd'?
Thanks,
Rob
John [H2O] wrote:
···
Hello,
I'm creating a web application that will take user input from a javascript
map to give me bounding coordinates (i.e. urcrnrlat, urcrnrlon, llcrnrlat,
llcrnrlon) and possibly a switch for polar projection. Other than that I
have no further information. Which projection is the most suitable to
handle anything from a 'global' plot to a zoom say over a state? I don't
see the zoom being too tight, but global projections are likely. I
personally prefer Equal Area, hence right now I'm working with 'aeqd', but
I seem to have problems if the plot is global with that projection.
Just looking for advice, opinions, and ideally examples if anyone has
created a similar function / module to use in a web environment.
Thanks!
-john
--
View this message in context: http://www.nabble.com/dynamic-basemap-tp20121594p21223766.html
Sent from the matplotlib - users mailing list archive at Nabble.com.
I just thought I would include some code so you can see what I mean.
from mpl_toolkits.basemap import Basemap
import matplotlib.pyplot as plt
import numpy as np
map=Basemap(projection='aeqd', lat_0=46, lon_0=-118.4, width=28300000,
height=28300000,
resolution='c', area_thresh=1000.)
map.drawcoastlines()
map.drawcountries()
map.fillcontinents(color='coral')
map.drawmapboundary()
plt.show()
Rob Frohne wrote:
···
Hi,
I think I am running into the same thing John is here. When you want to
display the whole earth in 'aeqd' mode, the projection needs to be onto a
circle. As it is, what is plotted is a square that just fits inside the
circle I want. Here is a link to a photo of the kind of projection I
want.
http://www.wm7d.net/az_proj/images/lon_anim_shaded.gif
Is there a way to get the whole earth plotted with 'aeqd'?
Thanks,
Rob
John [H2O] wrote:
Hello,
I'm creating a web application that will take user input from a
javascript map to give me bounding coordinates (i.e. urcrnrlat,
urcrnrlon, llcrnrlat, llcrnrlon) and possibly a switch for polar
projection. Other than that I have no further information. Which
projection is the most suitable to handle anything from a 'global' plot
to a zoom say over a state? I don't see the zoom being too tight, but
global projections are likely. I personally prefer Equal Area, hence
right now I'm working with 'aeqd', but I seem to have problems if the
plot is global with that projection.
Just looking for advice, opinions, and ideally examples if anyone has
created a similar function / module to use in a web environment.
Thanks!
-john
--
View this message in context: http://www.nabble.com/dynamic-basemap-tp20121594p21223795.html
Sent from the matplotlib - users mailing list archive at Nabble.com.
Hi Jeff,
I am an amateur radio operator, and I am writing a little application to
display where the major lobe of my antenna is pointing. I can control the
direction of my antenna with the computer, and it would be nice to have a
display of the whole world, as well as circles representing how far the
station I am talking to is, and radial lines showing the bearings. I'd like
to show the bearings along the edge of the map. For this type of map, a
circle is a much better boundary than a square, but if it has to be a
square, we should be able to make it a square bounding the circle, not the
other way around. I think I can even plot the station's location given data
I get from the Internet using this software. I am just learning Python, but
it appears to be ideal for what I'm doing.
Right now I'm trying to figure out how to get the Eclipse IDE I am trying
out to show me the source code for Basemap. 
Thanks for the expert help!
Rob
Jeff Whitaker wrote:
···
Rob Frohne wrote:
Hi,
I think I am running into the same thing John is here. When you want to
display the whole earth in 'aeqd' mode, the projection needs to be onto a
circle. As it is, what is plotted is a square that just fits inside the
circle I want. Here is a link to a photo of the kind of projection I
want.
http://www.wm7d.net/az_proj/images/lon_anim_shaded.gif
Is there a way to get the whole earth plotted with 'aeqd'?
Rob: No, you can't get the whole earth - the most you can get is the
cube that fits within it. I can look into adding that functionality for
the aeqd projection if there's a real use case. Note that there are
other whole-earth projections available (mollweide, vandergrinten,
robinson, sinuisoidal etc). These projections have much less distortion
far away from the center of the map than the azimuthal equidistant
does. Why do you want to use aeqd to plot the whole globe?
-Jeff
Thanks,
Rob
John [H2O] wrote:
Hello,
I'm creating a web application that will take user input from a
javascript
map to give me bounding coordinates (i.e. urcrnrlat, urcrnrlon,
llcrnrlat,
llcrnrlon) and possibly a switch for polar projection. Other than that I
have no further information. Which projection is the most suitable to
handle anything from a 'global' plot to a zoom say over a state? I don't
see the zoom being too tight, but global projections are likely. I
personally prefer Equal Area, hence right now I'm working with 'aeqd',
but
I seem to have problems if the plot is global with that projection.
Just looking for advice, opinions, and ideally examples if anyone has
created a similar function / module to use in a web environment.
Thanks!
-john
--
Jeffrey S. Whitaker Phone : (303)497-6313
Meteorologist FAX : (303)497-6449
NOAA/OAR/PSD R/PSD1 Email : Jeffrey.S.Whitaker@...259...
325 Broadway Office : Skaggs Research Cntr 1D-113
Boulder, CO, USA 80303-3328 Web : Jeffrey S. Whitaker: NOAA Physical Sciences Laboratory
------------------------------------------------------------------------------
_______________________________________________
Matplotlib-users mailing list
Matplotlib-users@lists.sourceforge.net
matplotlib-users List Signup and Options
--
View this message in context: http://www.nabble.com/dynamic-basemap-tp20121594p21225725.html
Sent from the matplotlib - users mailing list archive at Nabble.com.
Rob Frohne wrote:
Hi Jeff,
I am an amateur radio operator, and I am writing a little application to
display where the major lobe of my antenna is pointing. I can control the
direction of my antenna with the computer, and it would be nice to have a
display of the whole world, as well as circles representing how far the
station I am talking to is, and radial lines showing the bearings. I'd like
to show the bearings along the edge of the map. For this type of map, a
circle is a much better boundary than a square, but if it has to be a
square, we should be able to make it a square bounding the circle, not the
other way around. I think I can even plot the station's location given data
I get from the Internet using this software. I am just learning Python, but
it appears to be ideal for what I'm doing.
Right now I'm trying to figure out how to get the Eclipse IDE I am trying
out to show me the source code for Basemap. 
Thanks for the expert help!
Rob
Rob: I've updated basemap in SVN so that if neither a width/height or corner lat/lons are provided, the whole world is plotted in a circle with projection='aeqd'. If you can access SVN please try it out and let me know how it works for you. If not, I can provide you with a tarball.
The full-disk aeqd will not work right now with ellipsoids, only for perfect spheres. Is that a problem for you?
-Jeff
···
Jeff Whitaker wrote:
Rob Frohne wrote:
Hi,
I think I am running into the same thing John is here. When you want to
display the whole earth in 'aeqd' mode, the projection needs to be onto a
circle. As it is, what is plotted is a square that just fits inside the
circle I want. Here is a link to a photo of the kind of projection I
want.
http://www.wm7d.net/az_proj/images/lon_anim_shaded.gif
Is there a way to get the whole earth plotted with 'aeqd'?
Rob: No, you can't get the whole earth - the most you can get is the cube that fits within it. I can look into adding that functionality for the aeqd projection if there's a real use case. Note that there are other whole-earth projections available (mollweide, vandergrinten, robinson, sinuisoidal etc). These projections have much less distortion far away from the center of the map than the azimuthal equidistant does. Why do you want to use aeqd to plot the whole globe?
-Jeff
Thanks,
Rob
John [H2O] wrote:
Hello,
I'm creating a web application that will take user input from a
javascript
map to give me bounding coordinates (i.e. urcrnrlat, urcrnrlon,
llcrnrlat,
llcrnrlon) and possibly a switch for polar projection. Other than that I
have no further information. Which projection is the most suitable to
handle anything from a 'global' plot to a zoom say over a state? I don't
see the zoom being too tight, but global projections are likely. I
personally prefer Equal Area, hence right now I'm working with 'aeqd',
but
I seem to have problems if the plot is global with that projection.
Just looking for advice, opinions, and ideally examples if anyone has
created a similar function / module to use in a web environment.
Thanks!
-john
--
Jeffrey S. Whitaker Phone : (303)497-6313
Meteorologist FAX : (303)497-6449
NOAA/OAR/PSD R/PSD1 Email : Jeffrey.S.Whitaker@...259...
325 Broadway Office : Skaggs Research Cntr 1D-113
Boulder, CO, USA 80303-3328 Web : Jeffrey S. Whitaker: NOAA Physical Sciences Laboratory
------------------------------------------------------------------------------
_______________________________________________
Matplotlib-users mailing list
Matplotlib-users@lists.sourceforge.net
matplotlib-users List Signup and Options
Thanks Jeff! That is just wonderful! No need for a non-spherical earth
model for me.
Again, thanks!
Rob
Jeff Whitaker wrote:
···
Rob Frohne wrote:
Hi Jeff,
I am an amateur radio operator, and I am writing a little application to
display where the major lobe of my antenna is pointing. I can control
the
direction of my antenna with the computer, and it would be nice to have a
display of the whole world, as well as circles representing how far the
station I am talking to is, and radial lines showing the bearings. I'd
like
to show the bearings along the edge of the map. For this type of map, a
circle is a much better boundary than a square, but if it has to be a
square, we should be able to make it a square bounding the circle, not
the
other way around. I think I can even plot the station's location given
data
I get from the Internet using this software. I am just learning Python,
but
it appears to be ideal for what I'm doing.
Right now I'm trying to figure out how to get the Eclipse IDE I am trying
out to show me the source code for Basemap. 
Thanks for the expert help!
Rob
Rob: I've updated basemap in SVN so that if neither a width/height or
corner lat/lons are provided, the whole world is plotted in a circle
with projection='aeqd'. If you can access SVN please try it out and let
me know how it works for you. If not, I can provide you with a tarball.
The full-disk aeqd will not work right now with ellipsoids, only for
perfect spheres. Is that a problem for you?
-Jeff
Jeff Whitaker wrote:
Rob Frohne wrote:
Hi,
I think I am running into the same thing John is here. When you want
to
display the whole earth in 'aeqd' mode, the projection needs to be onto
a
circle. As it is, what is plotted is a square that just fits inside
the
circle I want. Here is a link to a photo of the kind of projection I
want.
http://www.wm7d.net/az_proj/images/lon_anim_shaded.gif
Is there a way to get the whole earth plotted with 'aeqd'?
Rob: No, you can't get the whole earth - the most you can get is the
cube that fits within it. I can look into adding that functionality for
the aeqd projection if there's a real use case. Note that there are
other whole-earth projections available (mollweide, vandergrinten,
robinson, sinuisoidal etc). These projections have much less distortion
far away from the center of the map than the azimuthal equidistant
does. Why do you want to use aeqd to plot the whole globe?
-Jeff
Thanks,
Rob
John [H2O] wrote:
Hello,
I'm creating a web application that will take user input from a
javascript
map to give me bounding coordinates (i.e. urcrnrlat, urcrnrlon,
llcrnrlat,
llcrnrlon) and possibly a switch for polar projection. Other than that
I
have no further information. Which projection is the most suitable to
handle anything from a 'global' plot to a zoom say over a state? I
don't
see the zoom being too tight, but global projections are likely. I
personally prefer Equal Area, hence right now I'm working with 'aeqd',
but
I seem to have problems if the plot is global with that projection.
Just looking for advice, opinions, and ideally examples if anyone has
created a similar function / module to use in a web environment.
Thanks!
-john
--
Jeffrey S. Whitaker Phone : (303)497-6313
Meteorologist FAX : (303)497-6449
NOAA/OAR/PSD R/PSD1 Email : Jeffrey.S.Whitaker@...259...
325 Broadway Office : Skaggs Research Cntr 1D-113
Boulder, CO, USA 80303-3328 Web : Jeffrey S. Whitaker: NOAA Physical Sciences Laboratory
------------------------------------------------------------------------------
_______________________________________________
Matplotlib-users mailing list
Matplotlib-users@lists.sourceforge.net
matplotlib-users List Signup and Options
------------------------------------------------------------------------------
_______________________________________________
Matplotlib-users mailing list
Matplotlib-users@lists.sourceforge.net
matplotlib-users List Signup and Options
--
View this message in context: http://www.nabble.com/dynamic-basemap-tp20121594p21234584.html
Sent from the matplotlib - users mailing list archive at Nabble.com.